React Tip: Only hyphenate really long words
For a client project I'm working on, I need a solution that would only hyphenate really long words, without allowing hyphens to appear all over the place. Hyphenation support in the browser are getting better, but it's still a long way to go.
Here is a simple solution I dreamt up (I actually dreamt this!).
import clsx from "clsx";
import { Fragment } from "react";
type HyphenatorProps = {
className?: string;
children?: string;
};
export function Hyphenator(props: HyphenatorProps) {
const words = props.children?.split(" ");
if (!words) return null;
return (
<div className={clsx(props.className, "")}>
{words.map((word, index) => (
<Fragment key={index}>
{word.length > 15 ? (
<span className="hyphens-auto">{word}</span>
) : (
word
)}
{index < words.length - 1 ? " " : ""}
</Fragment>
))}
</div>
);
}
export default function Home() {
return (
<main className="bg-yellow-200 w-[150px]">
<Hyphenator>Hello everyone world</Hyphenator>
<br />
<Hyphenator>Hello world thisisalongwordthatneedstobreak</Hyphenator>
</main>
);
}
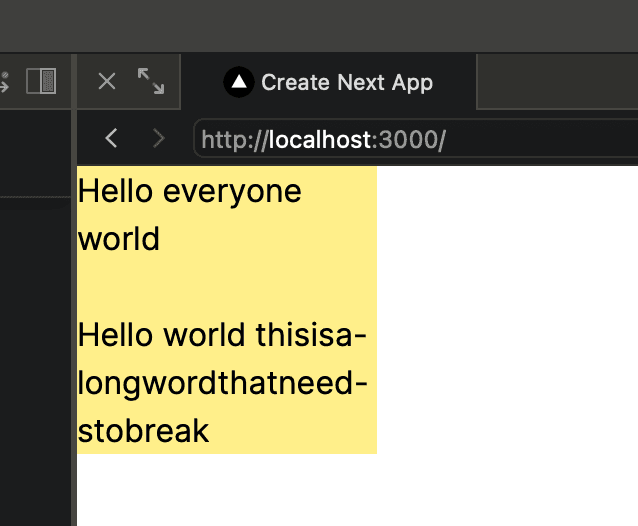
Now it's allowing hyphens if the word has more than 15 characters. One improvement to this solution, could be to see if we could approximate the length of the word compared to it's container (using em's and container queries maybe), and only break the word if it's longer than it's parent container.